A quick overview for iPhone programming
- Some people know everything about programming, some people know nothing.
- Programmers are lazy.
- Don't repeat yourself.
- We use objects and classes.
Let's look at some code
Objective C from one of my projects
Player *player = [Player sharedPlayer];
player.damageRange = NSMakeRange(1, 3);
player.maxHealth = 10;
player.health = 10;
player.money = 20;
Object Oriented Programming 101
Classes are great. We use them to model things.
For Example. Wanna make a chess game? Then you'll probably want to create a class for each of the types of pieces on the board, and a class for the board itself.
Objects are great because we can have lots of similar objects either inherit a lot of behavior from each other, for example. You may want to make a driving game. You can make a car object, then have other cars inherit from it and do more with the object.
Car object (psudo code)
class Car {
wheels = 4
speed = 10
drive(){
go_forwards( speed )
}
honk(){
say "beeep"
}
}
We can take the car object and extend it to make different types of cars without having to re-write large chunks of code.
Truck object
class Truck extends Car {
speed = 5
honk(){
say "HOOOOONKKKKK"
}
}
or a super fast car
Ortamobile object
class Ortamobile extends Car {
speed = 200
honk(){
say "neeeawwwnggggg"
}
}
iOS / Mac OS X we use objective-c. It's pretty crazy.
Like in cooking we have recipes, in programming these are called Header files. These files are a way of showing what the object will do, so you can quickly get an overview of responsibilities.
Car.h ( Obj-C Header )
#import < Foundation/Foundation.h>
@interface Car : NSObject(
// object variables go here
int speed;
int wheels;
)
// object functions ( or methods ) go outside
-(void)drive;
-(void)honk;
@end
After looking at this we can tell that a car has a speed variable, and a wheels variable, though we don't know what they are. Because we cannot set their values in the header.
Its complement is the implementation file.
To stretch the cooking metaphor this is the bit where we get our hands dirty and actually make things.
Car.m ( Obj-C Implementation )
#import "Car.h"
@implementation Car
-(id)init {
self = [super init];
speed = 10;
wheels = 4;
return self;
}
-(void) honk {
NSLog(@"Beep");
}
@end
NSLog() is like cout, or like println() it lets us write things out to the screen so we can see it's worked.
So in simple, a Class would look like this
Car.h ( Obj-C Header )
@interface Car : NSObject(
// object variables go here
)
// object functions go outside
// but before the @end
@end
and
Car.m ( Obj-C Implementation )
#import "Car.h"
@implementation Car
// actual methods go here
@end
In depth stuff. Sorry.
We’re working with a GUI, and that means you’ve got a lot more things to think about. So before we get started on what additional syntax we have to deal with iOS dev, we’re going to have to get some words right.
name |
meaning |
Objective-C |
Objective-C is a programming language, the ones you have heard of around here would be Ruby, Javascript and Coffeescript. They all have their plusses and negatives. |
Frameworks or Libraries |
A framework or library is a collection of classes made for you to use, in C++ you probably use std as your library, and in Java, well, I guess you used Java’s native classes. |
UIKit |
UIKit is the framework that gives us all the shiney touch controls, and provides access to everything from the accelerometer to the speakers. |
# |
This is a hash. This is a pound: £ |
When writing applications for iOS, you say you’re writing Objective-C with UIKit.
thought that was all?
So, that’s just the language. Let’s talk about the tools. We use two applications to make iOS apps. Those being XCode and Interface Builder.
name |
meaning |
XCode |
XCode is the tool you’ll be writing all your code in, you may have used Visual Studio or Eclipse before, it’s like that, but made by Apple. XCode is for handling all of the code aspects of writing your application; like your header (.h) and implementation (.m) files. |
Interface Builder |
Interface Builder is the tool that handles all the design think of it as you would Photoshop we’ll be using this a lot in our apps. |
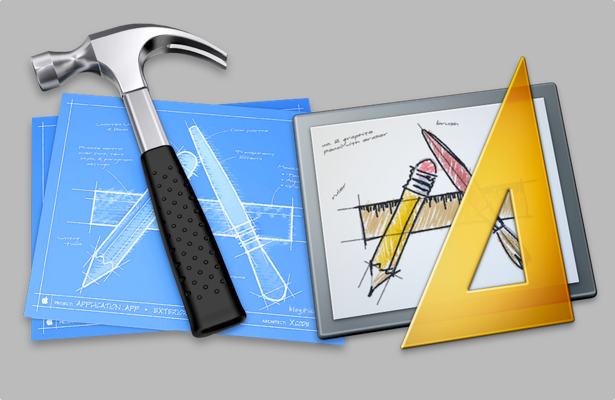
XCode 4
XCode is the program we use to make an iPhone app. You could think of this as the kitchen that holds all the tools that we’ll need to make something with.
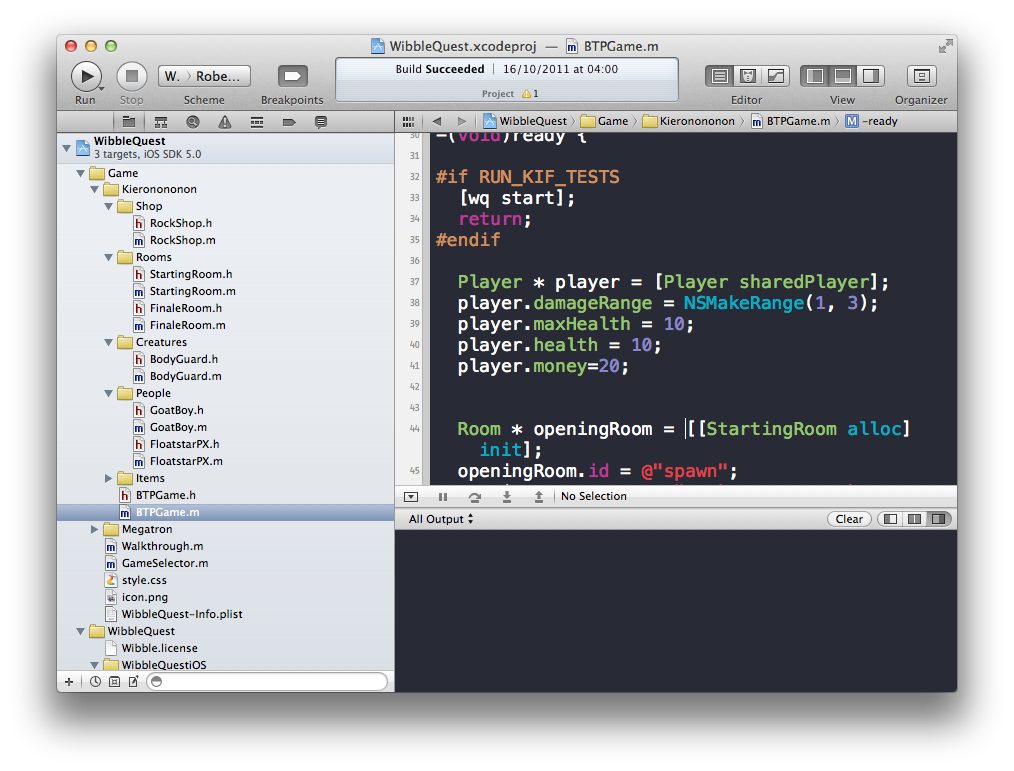
What now? Read these
Wonder how I learned this all? Apple have great documentation